前回の記事に引き続き、今回も仮想通貨botの開発状況をまとめていきます。
元々のコードからは、価格取得の流れがイマイチ理解できなかったので、初心にかえって「価格取得の方法」と「コンソールへの書き出し」を勉強し直しました。
これも、ccxtライブラリを使ったコードを書く学習の一環ですね。
残高を取得する関数
import ccxt import time # BitflyerのAPIキーとシークレットキーを設定 api_key = 'YourAPIKey' api_secret = 'YourAPISecret' exchange = ccxt.bitflyer({ 'apiKey': api_key, 'secret': api_secret, }) # 残高を取得する関数 def get_balance(): while True: try: # 残高情報を取得 balance = exchange.fetch_balance() return balance except ccxt.NetworkError as e: print(f"Network error: {e}") time.sleep(1) except ccxt.ExchangeError as e: print(f"Exchange error: {e}") time.sleep(1) except Exception as e: print(f"An unexpected error occurred: {e}") time.sleep(1) # 残高を取得してコンソールに表示 try: balance_info = get_balance() print("Balance Information:") print(balance_info) except Exception as e: print(f"An error occurred: {e}")
上記のコードの実行結果は以下の通り。
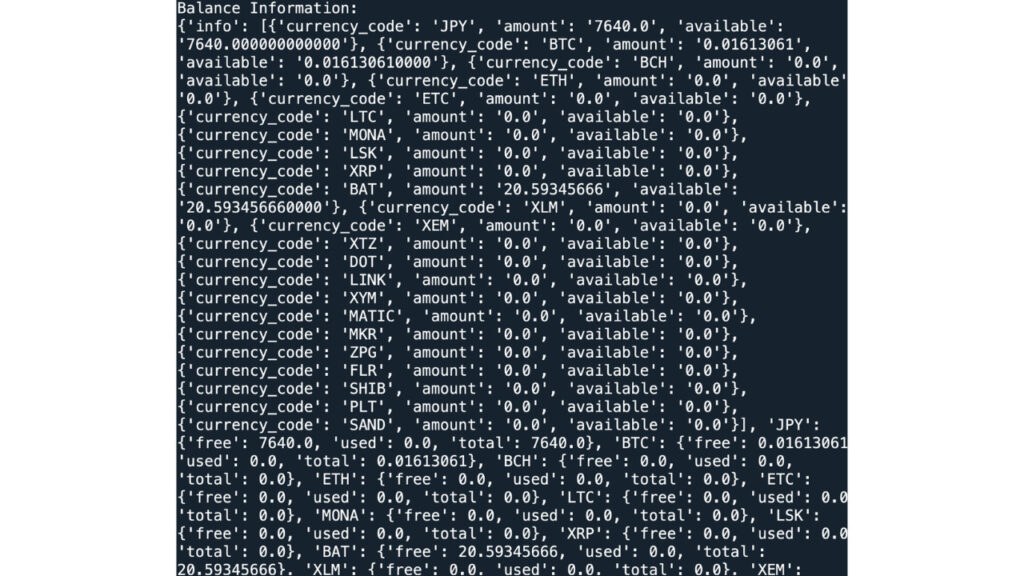
ものすごく多くの情報が表示されてしまうので、とても見にくい,,,。
ここは改良が必要ですね。
また、FX取引を利用するために「証拠金の残高」も獲得したいので、それに沿うようにコードを書き換えます。
0以上の残高と証拠金をコンソールに表示する
そこで「残高が0円以上の場合」と「FX証拠金の残高」を表示されるようにしました。
import ccxt import time # APIキーの設定 api_key = 'YourAPIKey' api_secret = 'YourAPISecret' exchange = ccxt.bitflyer({ 'apiKey': api_key, 'secret': api_secret, }) # 残高を取得する関数 def get_balance(): while True: try: balance = exchange.fetch_balance() return balance except ccxt.NetworkError as e: print(f"Network error: {e}") time.sleep(1) except ccxt.ExchangeError as e: print(f"Exchange error: {e}") time.sleep(1) except Exception as e: print(f"An unexpected error occurred: {e}") time.sleep(1) # JPY残高を取得する関数 def get_jpy_balance(): try: # ビットフライヤーのAPIを使用してJPY残高を取得 balance_info = get_balance() jpy_balance = float(balance_info['total']['JPY']) # Convert to float return jpy_balance except Exception as e: print(f"An error occurred while getting JPY balance: {e}") return None # JPY証拠金を取得する関数 def get_colla(): while True: try: colla = exchange.privateGetGetcollateral() return colla except ccxt.NetworkError as e: print(f"Network error: {e}") time.sleep(1) except ccxt.ExchangeError as e: print(f"Exchange error: {e}") time.sleep(1) except Exception as e: print(f"An unexpected error occurred: {e}") time.sleep(1) # メイン処理 try: # JPY残高を取得 jpy_balance = get_jpy_balance() # 取得した残高が0以上の場合にコンソールに表示 if jpy_balance is not None and jpy_balance > 0: print(f"JPY Balance: {jpy_balance}") # JPY証拠金を取得 colla_info = get_colla() jpy_colla = float(colla_info['collateral']) # Convert to float # 取得した証拠金が0以上の場合にコンソールに表示 if jpy_colla is not None and jpy_colla > 0: print(f"JPY Collateral: {jpy_colla}") except Exception as e: print(f"An error occurred: {e}")
上記のコードを実行して、「口座残高」と「証拠金」を表示させることができました。
口座残高・資産状況・FX証拠金を表示するコード
ChatGPTにあれこれ指示を出して「口座残高」「保有する仮想通貨の残高」「FX証拠金」を表示するコードを書かせることができました。
import ccxt import time # APIキーの設定 api_key = 'YourAPIKey' api_secret = 'YourAPISecret' exchange = ccxt.bitflyer({ 'apiKey': api_key, 'secret': api_secret, }) # 残高を取得する関数 def get_balance(): while True: try: balance = exchange.fetch_balance() return balance except ccxt.NetworkError as e: print(f"Network error: {e}") time.sleep(1) except ccxt.ExchangeError as e: print(f"Exchange error: {e}") time.sleep(1) except Exception as e: print(f"An unexpected error occurred: {e}") time.sleep(1) # JPY残高を取得する関数 def get_jpy_balance(): try: # ビットフライヤーのAPIを使用してJPY残高を取得 balance_info = get_balance() jpy_balance = float(balance_info['total']['JPY']) # Convert to float return jpy_balance except Exception as e: print(f"An error occurred while getting JPY balance: {e}") return None # 資産の価格が0円以上のものを取得する関数 def get_assets_above_zero(): try: assets_info = get_balance() assets = assets_info['total'] for asset, value in assets.items(): if value > 0 and asset != 'JPY': # JPY以外で0円以上の資産を表示 print(f"{asset}: {value}") except Exception as e: print(f"An error occurred while getting assets: {e}") # FX証拠金の残高を取得する関数 def get_fx_colla(): while True: try: fx_colla = exchange.privateGetGetcollateral() return float(fx_colla['collateral']) # Convert to float except ccxt.NetworkError as e: print(f"Network error: {e}") time.sleep(1) except ccxt.ExchangeError as e: print(f"Exchange error: {e}") time.sleep(1) except Exception as e: print(f"An unexpected error occurred: {e}") time.sleep(1) # メイン処理 try: # JPY残高を取得 jpy_balance = get_jpy_balance() # 取得した残高が0以上の場合にコンソールに表示 if jpy_balance is not None and jpy_balance > 0: print(f"JPY Balance: {jpy_balance}") # JPY以外の残高が0円以上の場合にコンソールに表示 get_assets_above_zero() # FX証拠金の残高を取得 fx_colla = get_fx_colla() # 取得したFX証拠金が0以上の場合にコンソールに表示 if fx_colla is not None and fx_colla > 0: print(f"FX Collateral: {fx_colla}") except Exception as e: print(f"An error occurred: {e}")
実行結果は以下の通り。
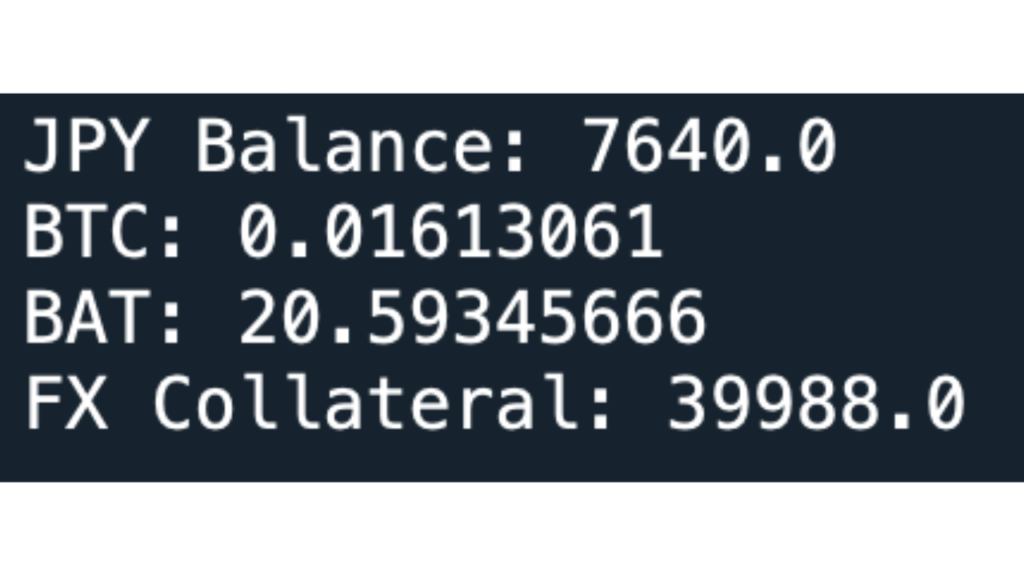
口座残高・保有する資産(BTCとBAT)・FX口座の証拠金が表示されているので、稼働成功です。
まとめ
今回は「口座の残高と証拠金を取得すること」と「それをコンソールに表示させること」ができるようになりました。
条件分岐の処理も少しこだわって試してみることもできました。
ccxtライブラリで様々な指示をスキップできるのでとても便利ですね。
次回もMMBotの開発を一つずつ進めていきます。