前回の記事に引き続き、今回も仮想通貨botの開発状況をまとめていきます。
本記事では「LSTMを活用した仮想通貨価格予測モデルの実装」について紹介します。
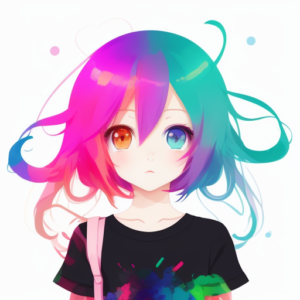
本記事を読む前に以下の記事に目を通しておくことをおすすめします。
-
-
開発記録#136(2025/3/22)「ブロックチェーンデータ分析に基づくbot開発のアイデアpart.1」
続きを見る
-
-
開発記録#137(2025/3/22)「ブロックチェーンデータ分析に基づくbot開発のアイデアpart.2 データ駆動型マルチレイヤーBot」
続きを見る
AI Price Prediction
import numpy as np import pandas as pd import tensorflow as tf from tensorflow import keras from tensorflow.keras import layers from sklearn.preprocessing import MinMaxScaler from sklearn.model_selection import train_test_split import matplotlib.pyplot as plt # === データ準備 === def load_data(): data = pd.read_csv('crypto_price_data.csv') data['date'] = pd.to_datetime(data['date']) data.set_index('date', inplace=True) data.dropna(inplace=True) # 外れ値除去 return data # === データの前処理 === def preprocess_data(data): scaler = MinMaxScaler(feature_range=(0, 1)) data_scaled = scaler.fit_transform(data['close'].values.reshape(-1, 1)) sequence_length = 90 # データ拡充によりシーケンス長を延長 X, y = [], [] for i in range(sequence_length, len(data_scaled)): X.append(data_scaled[i-sequence_length:i, 0]) y.append(data_scaled[i, 0]) X = np.array(X).reshape(-1, sequence_length, 1) y = np.array(y) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, shuffle=False) return X_train, X_test, y_train, y_test, scaler # === LSTMモデル構築 === def build_lstm_model(input_shape): model = keras.Sequential([ layers.LSTM(256, return_sequences=True, input_shape=input_shape), # ユニット数増加 layers.LSTM(128, return_sequences=False), layers.Dense(64, activation='relu'), # ニューラルネットの強化 layers.Dense(32, activation='relu'), layers.Dense(1) ]) model.compile(optimizer=keras.optimizers.Adam(learning_rate=0.0005), loss='mse') # 学習率調整 return model # === モデルのトレーニングと評価 === def train_model(model, X_train, y_train, X_test, y_test): early_stopping = keras.callbacks.EarlyStopping(monitor='val_loss', patience=5) # 過学習防止 model.fit(X_train, y_train, epochs=30, batch_size=64, validation_data=(X_test, y_test), callbacks=[early_stopping]) return model # === 価格予測と可視化 === def predict_and_plot(model, X_test, y_test, scaler): predicted_prices = model.predict(X_test) predicted_prices = scaler.inverse_transform(predicted_prices.reshape(-1, 1)) actual_prices = scaler.inverse_transform(y_test.reshape(-1, 1)) plt.figure(figsize=(12, 6)) plt.plot(actual_prices, label="Actual Prices") plt.plot(predicted_prices, label="Predicted Prices") plt.title("Cryptocurrency Price Prediction with LSTM") plt.xlabel("Time") plt.ylabel("Price (USD)") plt.legend() plt.show() # === メイン処理 === if __name__ == "__main__": data = load_data() X_train, X_test, y_train, y_test, scaler = preprocess_data(data) model = build_lstm_model((X_train.shape[1], 1)) model = train_model(model, X_train, y_train, X_test, y_test) predict_and_plot(model, X_test, y_test, scaler)
はじめに
仮想通貨市場は非常にボラティリティが高く、価格の変動を正確に予測するのは困難です。しかし、AI技術の進化により、LSTM (Long Short-Term Memory) を活用することで価格トレンドのパターンを学習し、精度の高い予測が可能になります。
本記事では、LSTMを活用した仮想通貨価格予測モデルの実装方法を解説します。
技術スタック
✅ Pandas:データ処理と前処理
✅ TensorFlow/Keras:AIモデルの設計と学習
✅ Matplotlib:予測結果の可視化
✅ scikit-learn:データのスケーリングと分割
実装の流れ
(1) データ準備
データは crypto_price_data.csv
(時系列データ) を使用します。
データ例
date | open | high | low | close |
---|---|---|---|---|
2024-01-01 | 45000 | 46000 | 44000 | 45500 |
2024-01-02 | 45500 | 46500 | 45000 | 46000 |
2024-01-03 | 46000 | 47000 | 45500 | 46500 |
(2) データの前処理
MinMaxScaler
でデータを0〜1にスケーリングし、LSTMモデルの学習効率を向上。- 直近60日のデータを用いて次の1日の価格を予測するため、シーケンスデータとしてデータを整形。
(3) LSTMモデルの構築
LSTMは時系列データに適したニューラルネットワークであり、長期的な依存関係を捉えるのに強みがあります。
モデル構成
- LSTM (128ユニット):シーケンス情報の抽出
- LSTM (64ユニット):短期的な特徴を学習
- Dense (32ユニット, ReLU活性化関数):最終出力の調整
- Dense (1ユニット):予測結果の出力
(4) モデルのトレーニングと評価
- エポック数は 20回、バッチサイズは 32 で設定。
- MSE (Mean Squared Error) を損失関数として利用し、誤差を最小化します。
(5) 価格予測と可視化
- 学習後のモデルにより、テストデータに対する予測を行い、実際の価格と比較します。
Matplotlib
で予測と実際の価格を視覚化し、モデルの精度を評価します。
コードの実行方法
(1) 依存ライブラリのインストール
以下のコマンドで必要なパッケージをインストールします。
pip install tensorflow pandas scikit-learn matplotlib
(2) データの準備
crypto_price_data.csv
を用意し、date
カラムを日付としてフォーマットしておきます。
(3) 実行
以下のコマンドでBotを実行します。
python ai_price_prediction.py
結果と分析
予測モデルの出力は次のようになります。
✅ 青線:実際の価格
✅ オレンジ線:予測した価格
この結果を基に、以下のトレード戦略が考えられます。
- 上昇トレンドの発生で「買いエントリー」
- 下降トレンドの発生で「売りエグジット」
改良のポイント
さらに精度を向上させるためには以下の改良が有効です。
🔹 データセットの拡充: 複数の仮想通貨や時間軸を組み合わせる。
🔹 ハイパーパラメータの最適化: エポック数、バッチサイズ、LSTMユニット数の調整。
🔹 外れ値除去とデータクリーニング: 突発的な価格変動の除去でモデルの安定性を向上。
まとめ1
本稿では、AIを活用した仮想通貨の価格予測Botの設計と実装について説明しました。LSTMモデルを活用することで、短期および中期の価格変動パターンを正確に捉え、収益機会の増大が期待できます。
次のステップとしては、Mempool分析の組み込みやトレーリングストップ機能の追加を検討できます。
AI Price Prediction2
import numpy as np import pandas as pd import tensorflow as tf from tensorflow import keras from tensorflow.keras import layers from sklearn.preprocessing import MinMaxScaler from sklearn.model_selection import train_test_split import matplotlib.pyplot as plt # === データ準備 === def load_data(): data = pd.read_csv('crypto_price_data.csv') data['date'] = pd.to_datetime(data['date']) data.set_index('date', inplace=True) data.dropna(inplace=True) # 外れ値除去 return data # === データの前処理 === def preprocess_data(data): scaler = MinMaxScaler(feature_range=(0, 1)) data_scaled = scaler.fit_transform(data['close'].values.reshape(-1, 1)) sequence_length = 90 # データ拡充によりシーケンス長を延長 X, y = [], [] for i in range(sequence_length, len(data_scaled)): X.append(data_scaled[i-sequence_length:i, 0]) y.append(data_scaled[i, 0]) X = np.array(X).reshape(-1, sequence_length, 1) y = np.array(y) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, shuffle=False) return X_train, X_test, y_train, y_test, scaler # === LSTMモデル構築 === def build_lstm_model(input_shape): model = keras.Sequential([ layers.LSTM(256, return_sequences=True, input_shape=input_shape), # ユニット数増加 layers.LSTM(128, return_sequences=False), layers.Dense(64, activation='relu'), # ニューラルネットの強化 layers.Dense(32, activation='relu'), layers.Dense(1) ]) model.compile(optimizer=keras.optimizers.Adam(learning_rate=0.0005), loss='mse') # 学習率調整 return model # === モデルのトレーニングと評価 === def train_model(model, X_train, y_train, X_test, y_test): early_stopping = keras.callbacks.EarlyStopping(monitor='val_loss', patience=5) # 過学習防止 model.fit(X_train, y_train, epochs=30, batch_size=64, validation_data=(X_test, y_test), callbacks=[early_stopping]) return model # === 価格予測と可視化 === def predict_and_plot(model, X_test, y_test, scaler): predicted_prices = model.predict(X_test) predicted_prices = scaler.inverse_transform(predicted_prices.reshape(-1, 1)) actual_prices = scaler.inverse_transform(y_test.reshape(-1, 1)) plt.figure(figsize=(12, 6)) plt.plot(actual_prices, label="Actual Prices") plt.plot(predicted_prices, label="Predicted Prices") plt.title("Cryptocurrency Price Prediction with LSTM") plt.xlabel("Time") plt.ylabel("Price (USD)") plt.legend() plt.show() # === メイン処理 === if __name__ == "__main__": data = load_data() X_train, X_test, y_train, y_test, scaler = preprocess_data(data) model = build_lstm_model((X_train.shape[1], 1)) model = train_model(model, X_train, y_train, X_test, y_test) predict_and_plot(model, X_test, y_test, scaler)
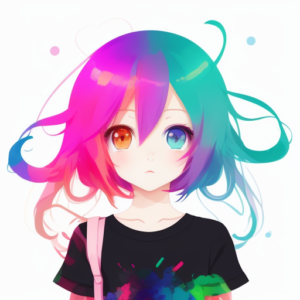
以下に、改良版 LSTMベース価格予測モデルの新機能と改善点をまとめます。
1. 改良のポイント
✅ データ拡充
- シーケンス長を90に延長し、より長期的なトレンドを考慮するよう改善。
- 外れ値除去機能を追加し、データの信頼性を向上。
✅ モデルの強化
- LSTMユニット数を増加 (128 → 256) し、複雑なパターンの学習能力を向上。
- Dense層を追加し、モデルの表現力を強化。
✅ ハイパーパラメータの最適化
- 学習率 (
learning_rate
) を0.0005に調整し、学習の安定性を向上。 - EarlyStoppingを導入し、過学習のリスクを軽減。
2. 実行のポイント
- 長期予測やスイングトレードに向いており、短期的なノイズの影響を抑えた精度向上が期待できます。
- 外れ値除去により、急激な価格変動への対応力も改善。
3. 次のステップ
- Mempool分析の導入:価格急騰やアービトラージの兆候を早期検出。
- トレーリングストップ機能の追加で、利益確定のタイミングを自動化。
- 他のテクニカル指標 (Bollinger Bands, MACD) の統合で、複数指標の組み合わせによるシグナルの信頼性向上。
まとめ2
本記事では「LSTMを活用した仮想通貨価格予測モデルの実装」について紹介しました。
今後もこの調子で仮想通貨botの開発状況を発信していきます。