前回の記事に引き続き、今回も仮想通貨botの開発状況をまとめていきます。
本記事では「暗号通貨のパンプ&ダンプスキームの検出」に関する論文をベースにbot開発の過程をまとめていきます。
Detecting Crypto Pump-and-Dump Schemes: A Thresholding-Based Approach to Handling Market Noisehttps://t.co/ctCJEV1MBs
— よだか(夜鷹/yodaka) (@yodakablog) March 22, 2025
🖥️ リアルタイム運用時の監視システム設計
次のステップでは、リアルタイム監視システムを構築し、以下の機能を実装します。
1. 監視システムのアプローチ
🔎 監視対象
✅ リアルタイム価格モニタリング:現在の価格が異常パターンに近づいた場合にアラート
✅ P&Dイベントの即時通知:Slack / Telegram への通知
✅ 取引状況のダッシュボード表示:Botの稼働状況、利益、損失などを視覚化
2. システム設計
📊 ダッシュボード ├── P&Dイベント検出状況 ├── 実行中のBotステータス ├── 現在の口座残高 ├── 取引履歴 └── アラート通知履歴
3. コード実装 (monitoring_system.py)
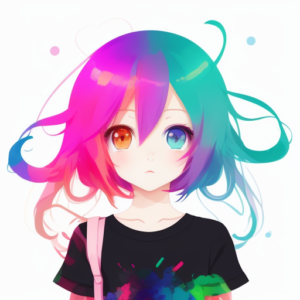
以下のコードは、ウェブソケットを使用してリアルタイムで仮想通貨の価格を監視し、異常な価格変動を検出してSlackに通知する、そしてFlaskを使ってダッシュボードを表示するPythonプログラムです。
import asyncio import json import websockets import requests import pandas as pd import matplotlib.pyplot as plt from flask import Flask, render_template # Flaskアプリケーションの設定 app = Flask(__name__) # Slack通知用 SLACK_WEBHOOK_URL = "YOUR_SLACK_WEBHOOK_URL" # データフレームの初期化 price_data = pd.DataFrame(columns=["timestamp", "symbol", "price"]) # WebSocket接続情報 (Bybit) API_URL = "wss://stream.bybit.com/realtime_public" SYMBOLS = ["BTCUSDT", "ETHUSDT"] # 異常検出パラメータ PRICE_THRESHOLD = 0.05 # 5%以上の急騰/急落でアラート # Slack通知機能 def send_alert(message): payload = {"text": message} requests.post(SLACK_WEBHOOK_URL, json=payload) # 価格モニタリング async def monitor_prices(): async with websockets.connect(API_URL) as websocket: # シンボルごとにデータ購読 for symbol in SYMBOLS: subscription_msg = { "op": "subscribe", "args": [f"ticker.{symbol}"] } await websocket.send(json.dumps(subscription_msg)) while True: try: response = await websocket.recv() data = json.loads(response) if 'topic' in data and 'data' in data: symbol = data['topic'].split('.')[-1] price = float(data['data']['last_price']) timestamp = pd.to_datetime("now") # データ追加 global price_data price_data = pd.concat([price_data, pd.DataFrame({ "timestamp": [timestamp], "symbol": [symbol], "price": [price] })]) # 価格異常の検出 recent_data = price_data[price_data['symbol'] == symbol] if len(recent_data) >= 2: price_change = (recent_data.iloc[-1]['price'] / recent_data.iloc[-2]['price']) - 1 if abs(price_change) > PRICE_THRESHOLD: alert_message = f"🚨 {symbol}の価格が{price_change*100:.2f}%変動しました" print(alert_message) send_alert(alert_message) except Exception as e: print(f"Error: {e}") await asyncio.sleep(5) # Flaskダッシュボード @app.route("/") def dashboard(): global price_data return render_template("dashboard.html", data=price_data.tail(20).to_dict(orient='records')) # メイン処理 def start_monitoring(): loop = asyncio.new_event_loop() asyncio.set_event_loop(loop) loop.run_until_complete(monitor_prices()) if __name__ == "__main__": from threading import Thread # Flaskアプリケーションをスレッドで実行 Thread(target=start_monitoring).start() app.run(debug=True, port=5000)
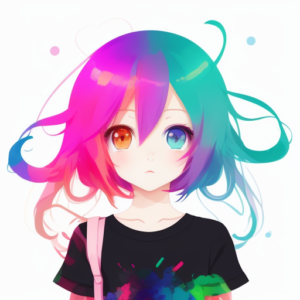
各セクションに分けて説明します。
インポートセクション
こちらでは、必要なライブラリをインポートしています。asyncio
は非同期プログラミングを支援、json
はJSONデータの処理、websockets
はWebSocket通信、requests
はHTTPリクエストを行うため、pandas
とmatplotlib.pyplot
はデータ処理と可視化、flask
はウェブアプリケーションの構築に使用されます。
Flaskアプリケーションの設定
Flask
クラスのインスタンスを生成して、アプリケーションの設定を行います。このインスタンスは、後ほど定義されるウェブサーバーの動作やルーティングの基盤となります。
Slack通知用の設定
SLACK_WEBHOOK_URL
には、SlackのWebhook URLを設定します。これにより、指定されたSlackチャネルに通知を送ることができます。
データフレームの初期化
price_data
という名前のpandasデータフレームを初期化して、時刻、シンボル、価格の列を用意します。このデータフレームは、収集した価格データの格納と分析に使用されます。
WebSocket接続とシンボルの設定
API_URL
はBybitのWebSocket APIのエンドポイント、SYMBOLS
は監視対象の仮想通貨ペアのリストです。これにより、リアルタイムで価格情報を取得し続けます。
異常検出パラメータ
PRICE_THRESHOLD
は価格変動のしきい値を設定しており、ここでは5%以上の価格変動があった場合にアラートを出すようになっています。
Slack通知機能
send_alert
関数は、引数で受け取ったメッセージをSlackに通知します。これはHTTP POSTリクエストを使用してSlackのWebhookを通じて行われます。
【参考1】Slack 通知を設定する
【参考2】【Slack】無料版でも簡単に作れるSlackアプリで通知Botを作る方法
【参考3】Slackアプリ開発を始めるときに全人類が知っておくべきこと
価格モニタリング関数
monitor_prices
は非同期関数で、WebSocketを通じて価格データを受け取り、データフレームに追加し、価格変動がしきい値を超えた場合にSlackにアラートを送信します。この関数は、WebSocketからのデータをリアルタイムで受け取り続けるため、無限ループ内で実行されます。
Flaskダッシュボード
dashboard
関数は、FlaskアプリケーションのルートURLにアクセスがあった際に呼び出され、dashboard.html
を用いて最新の価格データ20件を表示します。
メイン処理と並行処理の設定
プログラムのメイン部分であるif __name__ == "__main__":
内では、別スレッドでWebSocketの監視を開始し、メインスレッドではFlaskアプリケーションを実行します。これにより、ウェブサーバーが動作しながら非同期に価格監視が行われる設計になっています。
補足:ウェブソケット(WebSocket)とは
ウェブソケット(WebSocket)は、インターネット上での双方向通信を実現するための技術です。従来のHTTP通信では、クライアント(例えばウェブブラウザ)からサーバーへリクエストを送り、サーバーがレスポンスを返すという一方向の通信が基本でした。しかし、ウェブソケットを使用すると、一度接続が確立されれば、クライアントとサーバーの間でリアルタイムかつ双方向のデータ交換が可能になります。
ウェブソケットの特徴と利点
- 双方向通信: ウェブソケットは、サーバーとクライアントが同時に情報を送受信できる双方向通信をサポートしています。これにより、サーバーからクライアントへのプッシュ通知(データの自動送信)が可能になります。
- リアルタイム通信: ウェブソケットの通信は遅延が非常に少ないため、リアルタイムでのデータ交換が求められるアプリケーション(例えばライブチャット、オンラインゲーム、金融市場のトレーディングプラットフォームなど)に適しています。
- オーバーヘッドの削減: 従来のHTTPポーリングやロングポーリングに比べ、ウェブソケットは接続を維持する間、ヘッダ情報などのオーバーヘッドが非常に少なく済みます。これにより、ネットワークの負荷を軽減し、より効率的な通信が可能です。
- 全二重通信: クライアントとサーバーが同時にデータを送信できる全二重通信を実現します。
使用例
ウェブソケットは、ユーザーのインタラクションが頻繁に発生するウェブアプリケーションで広く使用されています。例えば、以下のような場面で活用されます。
- オンラインゲーム: プレイヤー間でのリアルタイムの状態同期を実現。
- チャットアプリケーション: メッセージの即時受信と送信。
- 金融アプリケーション: 株価や通貨の価格がリアルタイムで更新されるトレーディングプラットフォーム。
- スポーツライブ配信: 試合のスコアやプレイの状況をリアルタイムに更新。
ウェブソケットは、インターネット通信のパフォーマンスと効率を大幅に向上させる重要な技術であり、現代のウェブアプリケーション開発において不可欠な要素の一つとなっています。
【参考】ネットワークはなぜつながるのか 第2版 知っておきたいTCP/IP、LAN、光ファイバの基礎知識
4. Flaskテンプレートファイル (dashboard.html)
このHTMLファイルは、ウェブブラウザで表示するためのドキュメントで、「リアルタイム監視システム」として仮想通貨の価格データを表示するために設計されています。このファイルは、HTMLとCSS、そしてFlaskテンプレートの機能を利用して動的にデータを埋め込む構造を持っています。
<!DOCTYPE html> <html> <head> <title>リアルタイム監視システム</title> <style> body { font-family: Arial, sans-serif; margin: 20px; } h1 { color: #4CAF50; } table { width: 100%; border-collapse: collapse; } th, td { border: 1px solid #ddd; padding: 8px; text-align: center; } th { background-color: #4CAF50; color: white; } </style> </head> <body> <h1>🚨 リアルタイム監視システム 🚨</h1> <h2>価格データ</h2> <table> <tr> <th>タイムスタンプ</th> <th>シンボル</th> <th>価格 (USD)</th> </tr> {% for row in data %} <tr> <td>{{ row['timestamp'] }}</td> <td>{{ row['symbol'] }}</td> <td>{{ row['price'] }}</td> </tr> {% endfor %} </table> </body> </html>
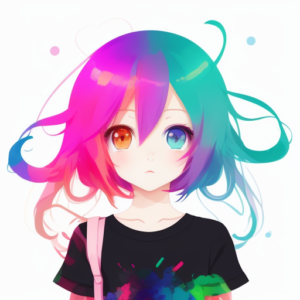
それぞれの部分について詳しく解説します。
DOCTYPE宣言
<!DOCTYPE html>
この宣言は、このHTMLファイルがHTML5規格に従っていることをブラウザに伝えます。これはHTML文書の最初に位置し、文書のタイプを定義します。
HTML要素
<html> ... </html>
<html>
タグは、HTML文書のルート要素です。これにより、文書全体の始まりと終わりを示します。
Headセクション
<head> <title>リアルタイム監視システム</title> <style> ... </style> </head>
<head>
タグ内では、文書のメタデータ(文書のタイトルやスタイルシートなど)を定義します。
<title>
タグは、ブラウザのタブに表示されるページのタイトルを設定します。<style>
タグ内にはCSSが記述されており、ページ内の要素のスタイリング(フォント、色、テーブルのスタイルなど)を指定しています。
Bodyセクション
<body> <h1>🚨 リアルタイム監視システム 🚨</h1> <h2>価格データ</h2> <table> ... </table> </body>
<body>
タグ内では、ユーザーに表示されるページのコンテンツが定義されています。
<h1>
と<h2>
タグでページの見出しとサブ見出しを設定しています。<table>
タグはデータを表形式で表示するために使用され、その中の<th>
タグで列のヘッダーを、<td>
タグでデータセルを定義しています。
Flaskテンプレート
{% for row in data %} <tr> <td>{{ row['timestamp'] }}</td> <td>{{ row['symbol'] }}</td> <td>{{ row['price'] }}</td> </tr> {% endfor %}
この部分は、Flaskのテンプレートエンジンを使用しています。{% for row in data %}
は、data
という変数が持つ各行に対して繰り返し処理を行うことを指示しており、その中の{{ row['timestamp'] }}
、{{ row['symbol'] }}
、{{ row['price'] }}
は、各行のタイムスタンプ、シンボル、価格データを動的にHTMLに埋め込むためのプレースホルダです。
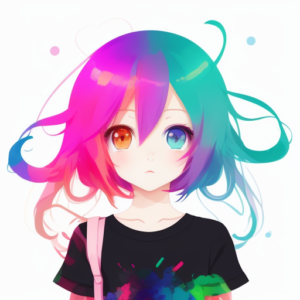
このファイル構造を通して、リアルタイムの価格監視データを綺麗に整形し、視覚的にも理解しやすい形でユーザーに提示することができます。
補足:Flaskとは?
Flaskは、Pythonで書かれた軽量なウェブアプリケーションフレームワークです。フレームワークとは、ある特定の目的を達成するために、あらかじめ用意されたツールやライブラリの集合のことを指します。Flaskは、ウェブサイトやウェブアプリケーションを作る際に必要な基本的な機能を提供し、拡張可能な構造であるため、小さなプロジェクトから大規模なアプリケーションまで幅広く対応できます。
Flaskの主な特徴
- シンプルさ: Flaskは「マイクロフレームワーク」とも呼ばれ、核となる機能に絞り込まれているため、学習が容易です。
- 拡張性: 必要に応じて機能を追加することができ、多くの追加パッケージや拡張機能を利用することができます。
- 柔軟性: データベースや他のツールとの組み合わせが自由で、開発者が好みに応じて様々な選択が可能です。
- 開発効率: Flaskは開発プロセスを迅速化し、小規模なアプリケーションを素早く簡単に構築するのに適しています。
- ルーティングシステム: URLから適切なビュー関数にリクエストを簡単に割り当てることができます。
Flaskはその単純さから初心者にも扱いやすく、プロトタイプの作成や小規模アプリの開発に特に適しています。また、大規模なアプリケーションにも拡張して使用することが可能です。データベースと組み合わせたり、RESTful APIを構築したり、動的なウェブページを提供するなど、多様な用途で利用されています。
参考
5. 実行方法&実行結果
1.必要なライブラリのインストール
pip install flask pandas matplotlib websockets requests
2.Slack Webhook URLをSLACK_WEBHOOK_URL
に設定
3.スクリプトの実行
python monitoring_system.py
- ダッシュボード表示
- ブラウザで以下にアクセス
👉http://localhost:5000
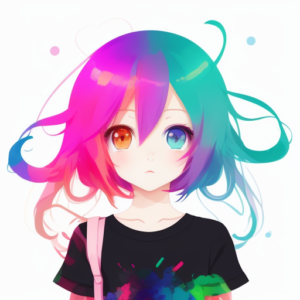
このコードを実行すると、以下のような結果が得られます。
- リアルタイム価格モニタリング: コードはBybitのAPIを通じて、指定された仮想通貨ペア(例えば、BTCUSDTとETHUSDT)の価格データをリアルタイムで監視します。WebSocketを使用しているため、市場の価格が更新されるたびにその情報を受け取り、システムに記録します。
- 価格変動アラート: 価格が前回のデータと比較して5%以上変動した場合、システムはその異常を検出し、設定されたSlackのWebhookを通じてアラートを送信します。この通知には、どの通貨ペアで何パーセント変動があったかの情報が含まれます。
- ダッシュボード表示: Flaskウェブアプリケーションを通じて、ブラウザでアクセス可能なダッシュボードが提供されます。このダッシュボードには、最新の20件の価格データが表形式で表示されます。これにより、最近の市場動向を迅速に確認できます。
- エラーハンドリング: 万が一WebSocket接続に問題が生じた場合やデータ受信中にエラーが発生した場合、コードはエラー情報を出力し、5秒後に再接続を試みます。これにより、一時的な接続問題に対してもシステムが稼働し続けます。
実行環境とインターフェース
- Webインターフェース: ダッシュボードはWebブラウザを通じてアクセス可能で、直感的なユーザーインターフェースで価格データを確認できます。
- Slack通知: Slackチャネルに送られるアラートにより、他の作業をしている間も市場の重要な変動を見逃さずに済みます。
6. 機能のポイント
✅ リアルタイム価格監視:Bybit WebSocket APIで即時データを取得
✅ Slack通知:異常が検出されるとアラートを送信
✅ ダッシュボード:Botの状況、価格、取引履歴を視覚的に確認
✅ エラーハンドリング:接続エラーや通信途絶に対応した再接続機能
7. 次のステップ
次の段階では、これまでに構築したシステムの統合、最終テスト、デプロイ手順の整備を行います。
デプロイには Docker + Kubernetes を活用し、Botのスケーラブルな運用環境を構築します。
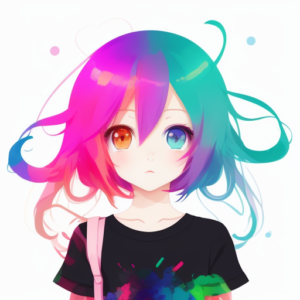
次回の記事では、「システム統合とデプロイ (Docker + Kubernetes)」についてまとめます。
-
-
開発記録#147(2025/3/25)「論文ベースのbot開発フローpart.9 システム統合とデプロイ (Docker + Kubernetes)」
続きを見る
おまけ:Flask以外のツール紹介
Flaskと同様にウェブアプリケーションを開発するために使える他のツールやフレームワークには、Django、Express.js、Ruby on Railsなどがあります。それぞれのフレームワークの特徴とFlaskとの比較を以下に述べます。
1. Django (Python)
特徴:
- DjangoはPythonで書かれた高レベルのWebフレームワークで、"batteries-included" アプローチを採用しています。これは、フレームワーク自体が多くの機能を内包していることを意味し、セキュリティ、データベース操作、ユーザ認証などの機能がすぐに使えます。
- より規模の大きい、複雑なウェブアプリケーションや企業レベルのプロジェクトに適しています。
Flaskとの比較:
- Flaskはマイクロフレームワークであり、必要最小限の機能のみを提供し、拡張が必要な場合は追加のパッケージを導入します。対照的にDjangoは多くの標準機能を提供するため、すぐに多機能なアプリケーションを開発できます。
2. Express.js (JavaScript - Node.js)
特徴:
- JavaScriptをサーバーサイドで実行するNode.jsのためのフレームワークです。非同期処理に優れ、リアルタイムアプリケーションやAPIサービスの開発に適しています。
- 軽量でありながら、ルーティング、ミドルウェアのサポートなど、柔軟なウェブアプリケーションの構築が可能です。
Flaskとの比較:
- Flaskと同様に、Expressもまた拡張性が高く、必要な機能をプラグインやミドルウェアを通じて追加することができます。ただし、JavaScriptの非同期ネイチャーはリアルタイムのウェブアプリケーションには特に適しており、これが大きな利点です。
3. Ruby on Rails (Ruby)
特徴:
- Ruby on RailsはRuby言語で書かれたフルスタックフレームワークで、Djangoと同様に多くの標準機能を提供します。"Convention over Configuration"(設定より規約)の原則により、必要な設定を最小限に抑え、素早くアプリケーション開発を進めることができます。
- 自動化されたテスト、セキュリティ機能が豊富で、開発プロセスが効率的です。
Flaskとの比較:
- Railsは初期のセットアップがDjango同様に重く、多くの機能が予め組み込まれているため、小さなプロジェクトにはオーバーキルとなる場合があります。Flaskはよりシンプルで軽量なため、小規模なプロジェクトや学習用プロジェクトに適しています。
これらの情報を表形式でまとめます。これにより、ブログ読者は各フレームワークの特徴を一目で比較できるようになります。
フレームワーク | 言語 | 特徴 | 適用例 | Flaskとの比較 |
---|---|---|---|---|
Flask | Python | 軽量、拡張性が高い | 小〜中規模のアプリ、プロトタイピング | - |
Django | Python | 多機能、セキュリティとデータベース管理が充実 | 中〜大規模のアプリ、企業用アプリケーション | より多機能だが重い |
Express.js | JavaScript | 非同期処理に優れ、軽量 | リアルタイムアプリ、APIサービス | JavaScriptベース、非同期処理に強い |
Ruby on Rails | Ruby | 規約重視、多機能 | スタートアップ向けの迅速なアプリ開発 | より規約重視、セットアップが重い |
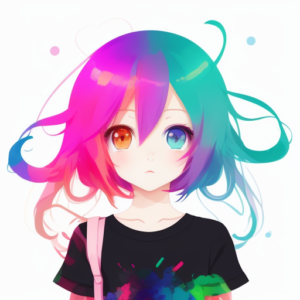
それぞれのフレームワークが異なるニーズやプロジェクトの規模によって、適切なツールを選ぶようにしましょう。
おまけ2:Slack
Slackは、ビジネスコミュニケーションを効率化するために設計されたプラットフォームです。1990年代に登場したIRC(Internet Relay Chat)を現代的にアップデートしたような形式で、テキスト、ビデオ、音声のメッセージング機能を提供し、チーム間のコミュニケーションやコラボレーションを容易にします。
Slackの主な特徴と機能
- チャンネル: ユーザーはプロジェクト、チーム、トピックごとに「チャンネル」と呼ばれるグループを作成できます。これにより、関連するディスカッションをテーマ別に整理して参加することができます。
- ダイレクトメッセージ: 二人以上のユーザーでプライベートに会話を行うことができる機能です。これにより、特定の議題について個別にコミュニケーションを取ることができます。
- 統合機能: Slackは数多くの外部サービスと統合可能です。例えば、Googleドライブ、Trello、GitHub、Asanaなどのツールと直接連携し、それらのサービスからの通知をSlack内で受け取ることができます。
- ファイル共有: チャンネルやダイレクトメッセージを通じてファイルを簡単に共有でき、参加者との間で必要なドキュメントや画像などをすぐに交換できます。
- 検索機能: Slackの強力な検索機能により、過去のメッセージや共有ファイルを簡単に見つけることができます。
- カスタマイズ: Slackでは、通知設定、外観、ショートカットなど、多くの側面をカスタマイズすることが可能です。
なぜSlackが好まれるか
- 集中と透明性: Slackは情報の分散を防ぎ、必要な情報が一箇所に集中することで、チームの透明性を高めます。
- 効率的なコミュニケーション: メールと比較して、よりスピーディーでインタラクティブなコミュニケーションを実現します。また、緊急時の迅速な対応が可能になります。
- リモートワークの支援: 分散したチーム間でも、まるで同じオフィスにいるかのようにコミュニケーションを取ることができます。
Slackは、特に情報を迅速に共有する必要があるビジネス環境や、リモートワークが多い現代の職場で非常に有効なツールとなっています。このように多機能でありながらも直感的な操作性を持つため、世界中の多くの企業や組織で広く採用されています。
類似ツール
Slackに似たコミュニケーションとコラボレーションのためのツールとして、以下のような主要な代替品があります。これらのツールはそれぞれ独自の特徴を持ちながら、チームのコミュニケーションやプロジェクトの管理を助けることを目的としています。
- Microsoft Teams
- 特徴: Microsoft Teamsは、Office 365の一部として提供され、ビデオ会議、チャット、ファイル共有などの機能を統合しています。特にMicrosoftの製品(Word, Excel, PowerPointなど)との連携が強く、企業内コミュニケーションとコラボレーションのための強力なツールです。
- 利点: Office 365ユーザーにとっては非常に便利で、追加コストなしで利用できる場合が多いです。
- Zoom
- 特徴: 元々はビデオ会議システムとして広く認知されていますが、チャット機能も提供しており、大規模なビデオコールやウェビナーに適しています。
- 利点: 高品質なビデオ会議が可能で、大人数でのコミュニケーションがスムーズに行えます。
- Google Chat and Google Meet
- 特徴: Google Workspace(旧称 G Suite)の一部として提供されるこれらのツールは、チャット、ビデオ会議、ドキュメント共有をシームレスに統合しています。
- 利点: Googleのエコシステム(Gmail, Google Docs, Google Driveなど)と深く統合されているため、これらのサービスを既に利用しているチームにとっては非常に便利です。
- Discord
- 特徴: 元々はゲーマー向けのプラットフォームとして開発されましたが、その高品質なボイスチャットとテキストチャットの機能により、多くの非ゲームコミュニティや企業でも使用されています。
- 利点: リアルタイムでの通信が非常にスムーズで、サーバーをカスタマイズして個別のニーズに合わせることができます。
- Mattermost
- 特徴: オープンソースの自己ホスティング可能なチャットサービスで、高度なセキュリティとプライバシーを求める企業向けに設計されています。
- 利点: サーバーを自社で管理できるため、データのプライバシーとセキュリティが厳格に保たれます。
これらのツールは、それぞれに異なる強みや特徴があり、組織のニーズに応じて選択することができます。例えば、Microsoftの製品を日常的に使用している企業はMicrosoft Teamsが最適である可能性が高く、ビデオ会議を頻繁に行う場合はZoomが強力な選択肢となります。